WSInterfaceFromLinkServer (C Function)
const char * WSInterfaceFromLinkServer(WSLinkServer s, int *err)
returns a C-style string that contains the IP address of the interface used by the link server s.
Details
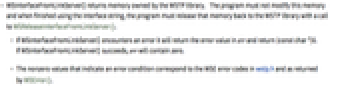
- WSInterfaceFromLinkServer() returns memory owned by the WSTP library. The program must not modify this memory and when finished using the interface string, the program must release that memory back to the WSTP library with a call to WSReleaseInterfaceFromLinkServer().
- If WSInterfaceFromLinkServer() encounters an error it will return the error value in err and return (const char *)0. If WSInterfaceFromLinkServer() succeeds, err will contain zero.
- The nonzero values that indicate an error condition correspond to the WSE error codes in wstp.h and as returned by WSError().
Examples
Basic Examples (1)
#include <stdlib.h> /* For malloc, free */
#include <string.h> /* For memset,memcpy */
#include "wstp.h"
void getInterfaceForLinkServer(WSLinkServer server)
{
char *buffer;
size_t length;
const char *interface;
int error;
interface = WSInterfaceFromLinkServer(server, &error);
if(interfaced == (const char *)0 || error != WSEOK)
{ /* Handle error */ }
length = strlen(interface);
buffer = (char *)malloc((length + 1) * sizeof(char));
if(buffer == (char *)0)
{ /* Handle out of memory condition */ }
memset((void *)buffer, 0, (length + 1) * sizeof(char));
memcpy((void *)buffer, (void *)interface, length * sizeof(char));
WSReleaseInterfaceFromLinkServer(server, interface);
/* .. do something with buffer... */
free(buffer);
return;
}