"Java" (External Evaluation System)
Details

- Java Version 9.0 and higher is supported.
- Java is a popular general-purpose programming language.
- Java does not require configuration to use with ExternalEvaluate.
ExternalEvaluate Usage

- ExternalEvaluate["Java",code] executes the code string in a Java REPL and returns the results as a Wolfram Language expression.
Data Types
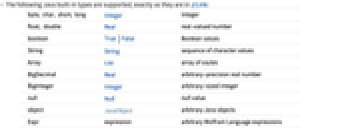
- The following Java built-in types are supported, exactly as they are in J/Link:
-
byte, char, short, long Integer integer float, double Real real-valued number boolean TrueFalse Boolean values String String sequence of character values Array List array of vaules BigDecimal Real arbitrary-precision real number BigInteger Integer arbitrary-sized integer null Null null value object JavaObject arbitrary Java objects Expr expression arbitrary Wolfram Language expressions
Usage Notes
- The Java runtime used by ExternalEvaluate is the same one used for J/Link operations like LoadJavaClass and JavaNew, allowing seamless interoperability between J/Link and ExternalEvaluate.
- Classes made visible to J/Link via its AddToClassPath function are visible to Java code in ExternalEvaluate.
- Java classes you define in ExternalEvaluate are automatically loaded into J/Link and can be used like any other Java classes.
Examples
open allclose allBasic Examples (5)
Evaluate 2+2 in Java and return the result:
Objects that have no natural representation are returned as JavaObject expressions, just as in J/Link:
Type > and select Java from the drop-down menu to get a code cell that uses ExternalEvaluate to evaluate:
Scope (2)
You can define a Java method without wrapping it in a full class definition. Note that such method definitions are always public and static so you can drop the "public static" prefix:
Method definitions return an ExternalFunction object that you can call:
public class Node {
private String data;
private Node next;
public Node(String data) {
this.data = data;
next = null;
}
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
public Node getNext() {
return next;
}
public void setNext(Node next) {
this.next = next;
}
}
Class definitions return J/Link JavaClass expressions that you can use directly in J/Link:
You can also call your new class directly from ExternalEvaluate: