WSBrowseCallbackFunction (C Function)
WSBrowseCallbackFunction
is a WSTP type that describes a function pointer to a function taking a WSENV object, a WSServiceRef object, an int object, a const char * object, and a void * object as arguments and returning void.
Details
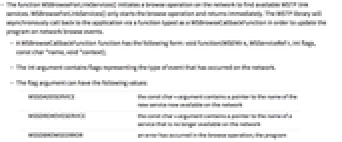
- The function WSBrowseForLinkServices() initiates a browse operation on the network to find available WSTP link services. WSBrowseForLinkServices() only starts the browse operation and returns immediately. The WSTP library will asynchronously call back to the application via a function typed as a WSBrowseCallbackFunction in order to update the program on network browse events.
- A WSBrowseCallbackFunction function has the following form: void function(WSENV e, WSServiceRef r, int flags, const char *name, void *context);
- The int argument contains flags representing the type of event that has occurred on the network.
- The flag argument can have the following values:
-
WSSDADDSERVICE the const char * argument contains a pointer to the name of the new service now available on the network WSSDREMOVESERVICE the const char * argument contains a pointer to the name of a service that is no longer available on the network WSSDBROWSEERROR an error has occurred in the browse operation; the program should use WSStopBrowsingForLinkServices() to terminate the browse operation; in addition, all cached service names should be removed; to receive further updates from the library regarding browse events, the program must call WSBrowseForLinkServices() again and initiate a new browse operation
Examples
Basic Examples (1)
#include "wstp.h"
void BrowseCallbackFunction(WSENV env, WSServiceRef ref, int flags, const char *serviceName, void *context);
WSServiceRef startBrowseOperation(WSENV e)
{
int apiResult;
WSServiceRef theRef;
apiResult = WSBrowseForLinkServices(e, BrowseCallbackFunction, NULL /* Use the default browse domain */, NULL /* no specific context object for this example */, &theRef);
if(apiResult != 0)
{ /* Handle the error. */ }
return theRef;
}
void BrowseCallbackFunction(WSENV env, WSServiceRef ref, int flags, const char *serviceName, void *context)
{
if(flags & WSSDADDSERVICE)
{ /* Register the service named in serviceName */ }
else if(flags & WSSDREMOVESERVICE)
{ /* Remove the service named in serviceName from the registry */ }
else if(flags & WSSDBROWSEERROR)
{ /* Stop the browse operation and clear the service name cache */ }
}