WSEndPacket (C Function)
int WSEndPacket(WSLINK link)
inserts an indicator in the expression stream that says the current expression is complete and is ready to be sent.
Details
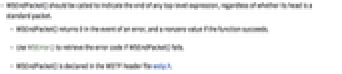
- WSEndPacket() should be called to indicate the end of any top-level expression, regardless of whether its head is a standard packet.
- WSEndPacket() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- Use WSError() to retrieve the error code if WSEndPacket() fails.
- WSEndPacket() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* send the expression {10, Times[3.56, 10], 1.3} to a link */
void f(WSLINK lp)
{
if(! WSPutFunction(lp, "List", 3))
{ /* unable to send the function List to lp */ }
if(! WSPutInteger32(lp, 10))
{ /* unable to send the integer 10 to lp */ }
if(! WSPutFunction(lp, Times, 2))
{ /* unable to send the function Times to lp */ }
if(! WSPutReal64(lp, 3.56))
{ /* unable to send the double 3.56 to lp */ }
if(! WSPutInteger32(lp, 10))
{ /* unable to send the integer 10 to lp */ }
if(! WSPutReal32(lp, 1.3))
{ /* unable to send the float 1.3 to lp */ }
if(! WSEndPacket(lp))
{ /* unable to send the end of packet message to lp */ }
}