"Python" (External Evaluation System)
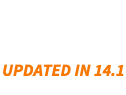
Details

- Python 3.4+ is supported.
- In most cases, the Wolfram Language will automatically provision one Python environment, and no manual user configuration is required. For advanced configuration, follow the instructions from the Configure Python for ExternalEvaluate workflow.
ExternalEvaluate Usage
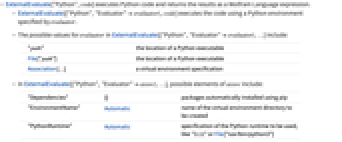
- ExternalEvaluate["Python",code] executes Python code and returns the results as a Wolfram Language expression.
- ExternalEvaluate[{"Python","Evaluator"evaluator},code] executes the code using a Python environment specified by evaluator.
- The possible values for evaluator in ExternalEvaluate[{"Python","Evaluator"evaluator},…] include:
-
"path" the location of a Python executable File["path"] the location of a Python executable Association[…] a virtual environment specification - In ExternalEvaluate[{"Python","Evaluator"assoc},…], possible elements of assoc include:
-
"Dependencies" {} packages automatically installed using pip "EnvironmentName" Automatic name of the virtual environment directory to be created "PythonRuntime" Automatic specification of the Python runtime to be used, like "3.11" or File["/usr/bin/python3"] "BaseDirectory" Automatic the directory of the created virtual environment - Possible settings for "type" in ExternalEvaluate["Python""type",code] include:
-
"Expression" attempt to convert to a Wolfram Language expression "String" give the raw string output by the external evaluator "ExternalObject" return the result as ExternalObject
Data Types
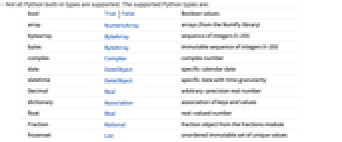
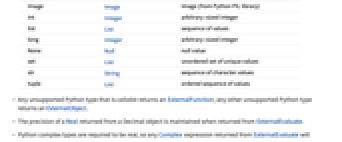
- Not all Python built-in types are supported. The supported Python types are:
-
bool TrueFalse Boolean values array NumericArray arrays (from the NumPy library) bytearray ByteArray sequence of integers 0-255 bytes ByteArray immutable sequence of integers 0-255 complex Complex complex number date DateObject specific calendar date datetime DateObject specific date with time granularity Decimal Real arbitrary-precision real number dictionary Association association of keys and values float Real real-valued number Fraction Rational fraction object from the fractions module frozenset List unordered immutable set of unique values Image Image image (from Python PIL library) int Integer arbitrary-sized integer list List sequence of values long Integer arbitrary-sized integer None Null null value set List unordered set of unique values str String sequence of character values tuple List ordered sequence of values - Any unsupported Python type that is callable returns an ExternalFunction; any other unsupported Python type returns an ExternalObject.
- The precision of a Real returned from a Decimal object is maintained when returned from ExternalEvaluate.
- Python complex types are required to be real, so any Complex expression returned from ExternalEvaluate will have real-valued components.
Supported External Operations
- ExternalOperation["Eval","code"] represents an external evaluation of "code".
- ExternalOperation["Eval","code",assoc] represents an external evaluation of "code" with parameters given by assoc.
- ExternalOperation["Call",func,arg1,arg2,…] calls the function func with the given arguments arg1, arg2, ….
- ExternalOperation["GetAttribute",obj,"attr"] gets the attribute "attr" of obj.
- ExternalOperation["SetAttribute",obj,"attr",val] sets the attribute "attr" of obj to the given value val.
- ExternalOperation["GetItem",obj,"item"] gets the item "item" of obj.
- ExternalOperation["SetItem",obj,"item",val] sets the item "item" of obj to the given value val.
- ExternalOperation["Import","path"] imports the module "path".
- ExternalOperation["Import","path","attr"] imports the module "path", then gets the attribute "attr" from the module.
- ExternalOperation["Cast",obj,"type"]casts obj to the given "type".
Examples
open allclose allBasic Examples (3)
Evaluate in Python and return the result:
Create a list in Python and return the result:
Type > to get a Python code cell that uses ExternalEvaluate to evaluate:
Create a list with exponentials and logarithms:
Create a list with the days of the week:
Use the File wrapper to execute code contained in a file:
Deploy code using CloudDeploy and then run the code directly from a CloudObject:
Use a URL wrapper to directly run code hosted online:
An Association can be used to automatically provision a Python virtual environment:
The Python virtual environment was created under $UserBaseDirectory:
Scope (49)
Evaluate a Boolean statement in Python and return the result:
Create a byte array in Python and return its equivalent in the Wolfram Language:
Another way to work with a byte array in Python:
Python list, tuple, set and frozenset are converted to List:
Python dict are converted to Association:
When using a Python higher than 3.7, dictionary keys are ordered, just like Association:
Create a Complex number in Python and return the result:
Fractions are automatically converted to Rational:
Decimals are converted to Real:
Datetime objects are automatically converted:
NumPy arrays are automatically converted to NumericArray:
Pandas DataFrames are automatically converted to Dataset:
PIL images are automatically converted to Image:
Generators are automatically converted to List:
Any Python object that is iterable will be automatically converted to a List unless an explicit conversion method is implemented:
String templates can be used to insert Wolfram Language expressions into Python code. Set two variables:
The expression x^2+y^2 is evaluated in the Wolfram Language, and the result is converted and inserted into the Python code string:
Session Options (12)
"ReturnType" (3)
For the Python evaluation system, the default return type is "Expression":
Numbers, strings, lists and associations are automatically imported for the "Expression" return type:
The return type of "String" returns a string of the result by calling the Python function repr:
"Evaluator" (5)
Evaluate Python code using a specified "Evaluator":
An Association can be used to automatically provision a Python virtual environment:
"Dependencies" can be a list of strings that specify Python packages, using pip conventions to specify versions:
The File wrapper can also be used in order to specify "Dependencies" for an evaluator:
Specify an "EnvironmentName" to customize the virtual environment folder name:
Specify a "BaseDirectory" to change the installation directory:
"PythonRuntime" can be used to download a specific version:
Use the File wrapper to use your own Python installation:
Command Options (11)
"Command" (4)
When only a string of Python code is provided, the command is directly executed:
The above is equivalent to writing the command using this form:
Use a File wrapper to run the code in a file:
The above is equivalent to writing the command using this form:
Use a URL wrapper to directly run code hosted online:
The above is equivalent to writing the command using this form:
Put code in a CloudObject:
Evaluate directly from the cloud:
The above is equivalent to writing the command using this form:
"ReturnType" (1)
"Arguments" (2)
Use "Arguments" to call a Python function with arguments:
When a non-list argument is provided, a single argument is passed to the function:
If you need to pass a list as the first argument, you must wrap it with an extra list explicitly:
You can define a function inside "Command" and directly call it with "Arguments":
The same result can be achieved by using a Rule:
You can also pass arguments by creating an ExternalFunction:
"TemplateArguments" (3)
When running a command, you can inline a TemplateExpression:
You can explicitly fill TemplateSlot using "TemplateArguments":
When a non-list argument is provided, a single template argument is passed to the template:
If you need to pass a list as the first argument, you must wrap it with an extra list explicitly:
You can name template slots and use an Association to pass named arguments to the template:
External Operations (16)
"Eval" (1)
Run an ExternalOperation that represents arbitrary code evaluation in Python:
"Call" (3)
Define an ExternalOperation that creates a function in Python:
Define a function call by running the ExternalOperation "Call":
Run the external operation representing the function call using ExternalEvaluate:
Any argument of the "Call" operation can be an ExternalOperation:
Arguments can also be passed directly in ExternalEvaluate by doing the following:
The result is equivalent of running the following Python code:
Create an ExternalFunction for a Python function:
Call the function by running the operation "Call":
The same result can be achieved by doing the following:
Create an ExternalObject for a Python function:
Call the function by running the operation "Call":
The same result can be achieved by doing the following:
Alternatively, you can use ExternalObject subvalues:
"GetAttribute" (1)
Start a Python session to work with dates:
Return an ExternalObject for a datetime object:
Extract the year attribute by using "GetAttribute":
The same can be done using an ExternalObject subvalue:
For the Python evaluator, "GetAttribute" is the default operation, and ExternalOperation can be omitted:
The result is equivalent to running the following Python code:
"SetAttribute" (1)
"GetItem" (2)
Create a Python dictionary and return it as an ExternalObject:
Extract the "name" item by using "GetItem":
The result is equivalent to running the following Python code:
Create an ExternalOperation that represents a dictionary:
Create a new ExternalOperation to get the item "name":
Run the operation using ExternalEvaluate:
"SetItem" (1)
Create an ExternalObject with a dictionary:
Check that the item was set to "joe":
Get the whole dictionary as an association, by using Expression:
"Import" (6)
Define an ExternalObject that imports the "datetime" module:
Return the module as an ExternalObject:
Define an ExternalObject that imports the date from the "datetime" module:
Call the operation with arguments using ExternalEvaluate:
Define an ExternalObject that imports the "datetime" module:
Use an ExternalFunction to call the method:
Define an ExternalObject that imports the fractions module:
Initialize the fraction by using ExternalObject subvalues:
The same can be done with the following syntax:
Define an "Import" operation that imports "ip_address":
Create an address instance using ExternalEvaluate:
Use String as the return type to show the Python string version of the instance:
Define an "Import" operation that imports "ip_address":
Create an address instance using another ExternalOperation:
Execute the operation in ExternalEvaluate:
"Cast" (1)
Create an ExternalObject that represents the current date:
Use "Expression" to return the object as a Wolfram Language expression:
The Cast operation can also run in ExternalObject subvalues:
The symbol Expression is a shortcut for the same:
Return the object as a string:
The symbol String is a shortcut for the same:
Return the object as an ExternalObject:
The symbol ExternalObject is a shortcut for the same:
The same can be achieved by using "ReturnType" in ExternalEvaluate:
Applications (3)
Properties & Relations (1)
You can use to return expressions from Python:
Expressions automatically evaluate after they are transferred in a kernel.
Use Hold to control evaluation:
Possible Issues (1)
Generators are automatically converted to List:
Returning an infinite generator will block your kernel forever: