"Image" (Net Encoder)
NetEncoder["Image"]
represents an encoder that converts a 2D image to a rank-3 tensor of pixel values.
NetEncoder[{"Image",size}]
represents an encoder that resizes the input image to size.
NetEncoder[{"Image",{width,height}}]
represents an encoder that resizes the input image to the specified dimensions.
NetEncoder[{"Image",size,"param"val,…}]
represents an encoder with specific parameters for preprocessing.
Details
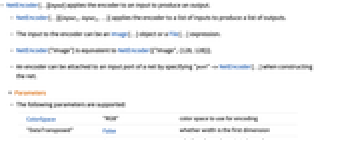
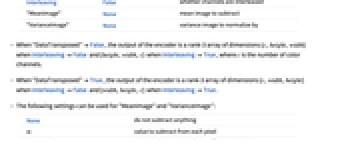
- NetEncoder[…][input] applies the encoder to an input to produce an output.
- NetEncoder[…][{input1,input2,…}] applies the encoder to a list of inputs to produce a list of outputs.
- The input to the encoder can be an Image[…] object or a File[…] expression.
- NetEncoder["Image"] is equivalent to NetEncoder[{"Image",{128,128}}].
- An encoder can be attached to an input port of a net by specifying "port"->NetEncoder[…] when constructing the net.
- The following parameters are supported:
-
ColorSpace "RGB" color space to use for encoding "DataTransposed" False whether width is the first dimension Interleaving False whether channels are interleaved "MeanImage" None mean image to subtract "VarianceImage" None variance image to normalize by Method "Stretch" how to conform the size Resampling Automatic resampling method Alignment Center how to align the image for Method"Fit" or "Fill" Padding Black padding scheme for Method"Fit" - Possible values for Method are:
-
"Stretch" stretch the image to fit by resampling "Fit" fit the whole image; keep the aspect ratio; pad if necessary "Fill" fit the smaller dimension; crop the other if necessary - The Interleaving and "DataTransposed" options affect the output shape in the following way:
- The following settings can be used for "MeanImage" and "VarianceImage":
-
None do not subtract anything m value to subtract from each pixel {m1,m2,…} values to be subtracted from different channels Image[…] overall image to subtract - Pixels are normalized to lie between 0 and 1 before doing subtraction.
Parameters
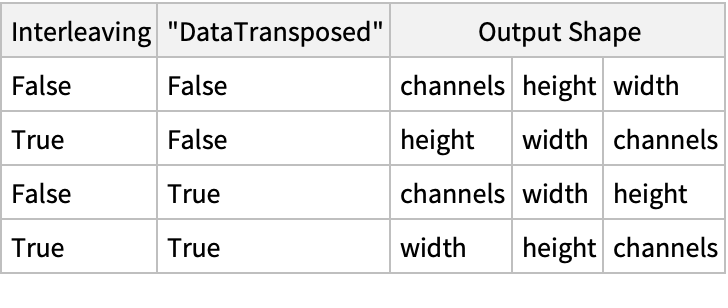
Examples
open allclose allBasic Examples (1)
Scope (1)
NetEncoder["Image"] can encode either File or Image objects. Create an image encoder:
Apply the encoder to a File object:
Apply the encoder to an Image object:
Parameters (12)
ColorSpace (1)
Set the ColorSpace of the output image to "Grayscale":
The output only has one channel dimension, appropriate for grayscale images.
"DataTransposed" (2)
With "DataTransposed"False, the height is the first dimension in the output:
With "DataTransposed"True, the width is the first dimension in the output:
With "DataTransposed"True and InterleavingTrue, the image can be scaled to a fixed height and a varying width:
Interleaving (2)
With InterleavingFalse, the channel dimension of the output is the first dimension in the dimensions list:
With InterleavingTrue, the channel dimension of the output is the last dimension in the dimensions list:
With InterleavingTrue, the image can be scaled to a fixed width and a varying height:
"MeanImage" (1)
"VarianceImage" (1)
Method (2)
Resampling (1)
Padding (1)
Properties & Relations (2)
When applying an image encoder to a list of File objects, NetEncoder will attempt to parallelize the computation on multicore processors. Create a list of 64 images:
Show the number of available processors:
Map the encoder over the images:
To exploit parallelism, apply the encoder to a list of File objects directly rather than with Map:
NetTrain will automatically try to attach an encoder when a net is not fully specified. Automatic attachment of an image encoder: