Use the Wolfram Client Library for Python to start an authenticated session with the Wolfram Cloud.
Using a Wolfram Notebook...
Verify that the Wolfram Client Library for Python is installed
Open a terminal window and enter pip show wolframclient to check if the library is installed:
> pip show wolframclient
Name: wolframclient
Version: 1.1.3
Summary: A Python library with various tools to interact with the Wolfram Language and the Wolfram Cloud.
Home-page: https://www.wolfram.com/
Author: Wolfram Research
Author-email: support@wolfram.com
License: MIT
Location: \Users\person\appdata\local\programs\python\python36\lib\site-packages
Requires: pip, numpy, pytz, requests, aiohttp, oauthlib, pyzmq
Required-by: wolframwebengine
- If the Wolfram Client Library (WCL) for Python is not installed, follow the setup instructions in the Install the Wolfram Client Library for Python workflow.
Generate a Secured Authentication Key
With the Wolfram Language, use GenerateSecuredAuthenticationKey to create unique keys to connect to a Wolfram Cloud session. You may be prompted to connect to the Wolfram Cloud first:
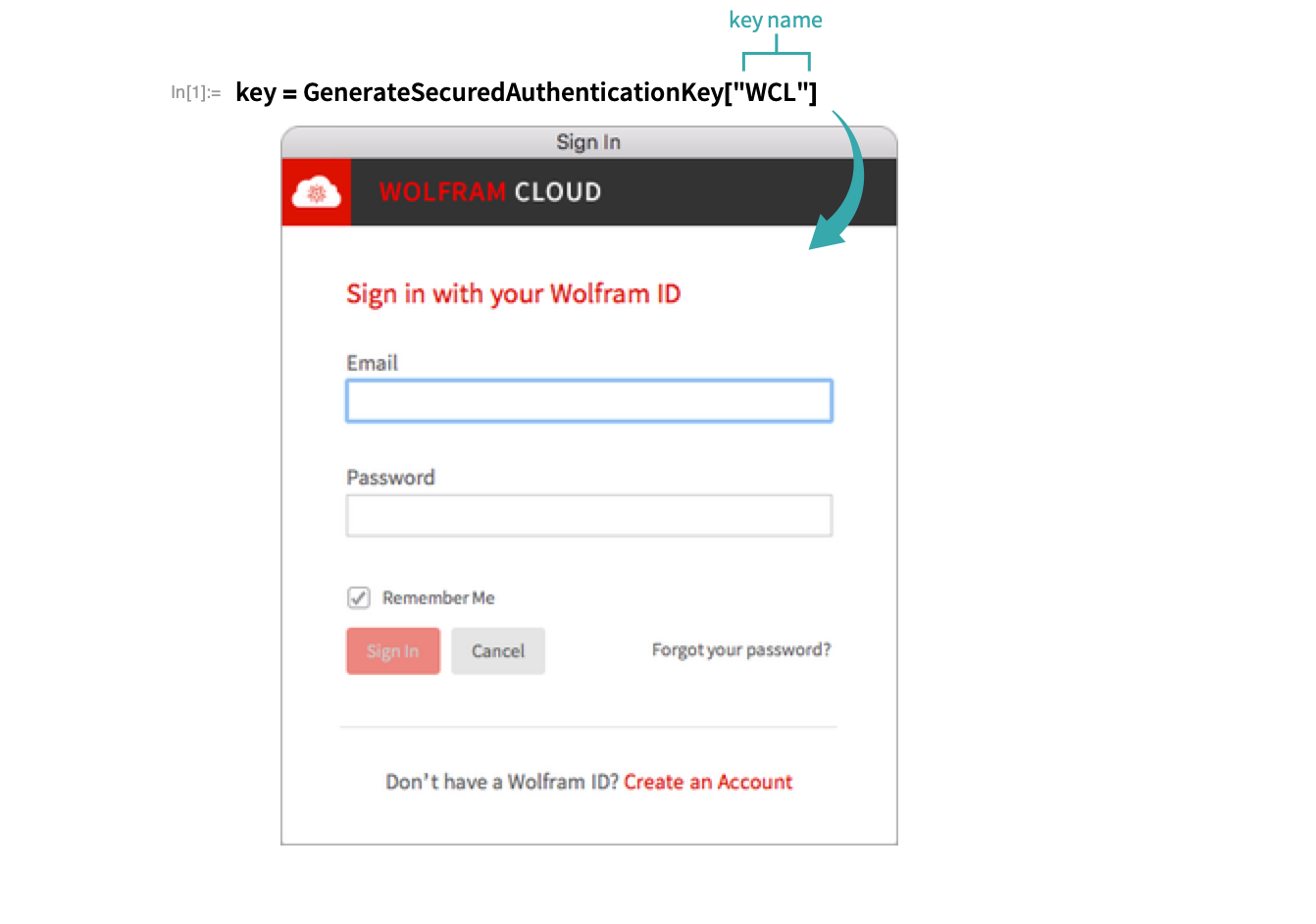
The output is a key object:

Use this object to get the consumer credentials as strings:
Import the following Wolfram Client Library classes
Initialize a Python cell in a notebook with :
Import the and
modules:
- WolframCloudSession is the main module to interact with the Wolfram Cloud.
- WolframCloudSession typically connects to the Wolfram Public Cloud.
- SecuredAuthenticationKey is a module storing consumer key and secret used during authentication.
Start an authenticated session
Copy and paste the previously generated consumer credentials into the associated Python function:
Initialize a cloud session using the default configuration targeting the Wolfram Public Cloud:
Check to verify that you are now authorized to use the Wolfram Cloud:
Execute a Wolfram Language evaluation in the cloud, returning the result to Python:
Using a Jupyter Notebook...
Verify that the Wolfram Client Library for Python is installed
Open a terminal window and enter pip show wolframclient to check if the library is installed:
> pip show wolframclient
Name: wolframclient
Version: 1.1.3
Summary: A Python library with various tools to interact with the Wolfram Language and the Wolfram Cloud.
Home-page: https://www.wolfram.com/
Author: Wolfram Research
Author-email: support@wolfram.com
License: MIT
Location: \Users\person\appdata\local\programs\python\python36\lib\site-packages
Requires: pip, numpy, pytz, requests, aiohttp, oauthlib, pyzmq
Required-by: wolframwebengine
- If the Wolfram Client Library (WCL) for Python is not installed, follow the setup instructions in the Install the Wolfram Client Library for Python workflow.
Generate a Secured Authentication Key
With the Wolfram Language, use GenerateSecuredAuthenticationKey to create unique keys to connect to a Wolfram Cloud session. You may be prompted to connect to the Wolfram Cloud first:
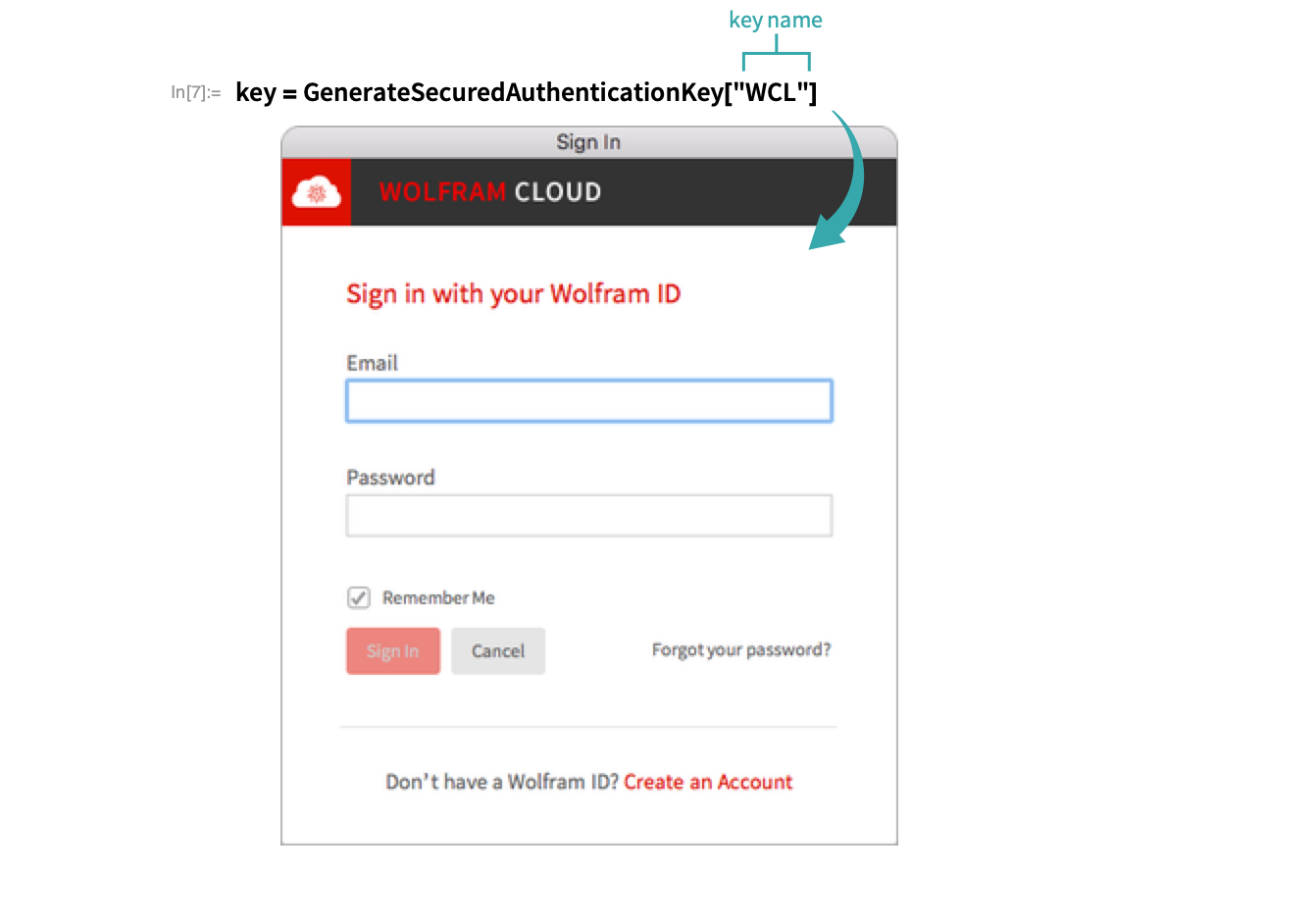
The output is a key object:

Use this object to get the consumer credentials as strings:
Import the following Wolfram Client Library classes
Import the and
modules:
- WolframCloudSession is the main module to interact with the Wolfram Cloud.
- WolframCloudSession typically connects to the Wolfram Public Cloud.
- SecuredAuthenticationKey is a module storing consumer key and secret used during authentication.
Start an authenticated session
Copy and paste the previously generated consumer credentials into the associated Python function:
Initialize a cloud session using the default configuration targeting the Wolfram Public Cloud:
Check to verify that you are now authorized to use the Wolfram Cloud:
Execute a Wolfram Language evaluation in the cloud, returning the result to Python: