EntityStore
EntityStore["type"]
represents an empty entity store for entities of type "type".
EntityStore["type"data]
represents an entity store for entities of type "type" with properties and values defined by data.
EntityStore[{tspec1,tspec2,…}]
represents an entity store for entities of multiple types.
EntityStore[RelationalDatabase[…]]
constructs an entity store from the schema of an external database.
EntityStore[{tspec1,tspec2,...},dbspec]
constructs an entity store by mapping table names in the database specified by dbspec to types as specified by the tspeci.
Details and Options
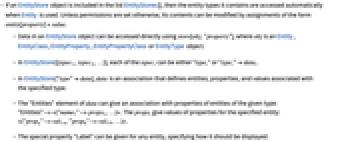
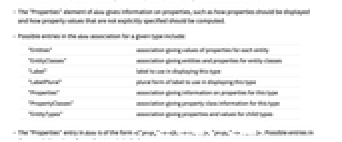
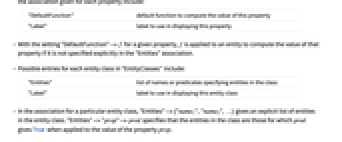
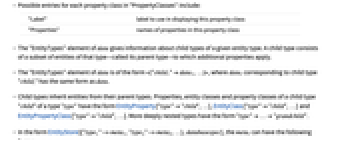
- If an EntityStore object is included in the list EntityStores[], then the entity types it contains are accessed automatically when Entity is used. Unless permissions are set otherwise, its contents can be modified by assignments of the form entity[property]=value.
- Data in an EntityStore object can be accessed directly using store[obj,"property"], where obj is an Entity, EntityClass, EntityProperty, EntityPropertyClass or EntityType object.
- In EntityStore[{tspec1,tspec2,…}], each of the tspeci can be either "typei" or "typei"datai.
- In EntityStore["type"data], data is an association that defines entities, properties, and values associated with the specified type.
- The "Entities" element of data can give an association with properties of entities of the given type: "Entities"-><"name1"->props1,… >. The propsi give values of properties for the specified entity: <"propa"->val1a,"propb"->val1b,… >.
- The special property "Label" can be given for any entity, specifying how it should be displayed.
- The "Properties" element of data gives information on properties, such as how properties should be displayed and how property values that are not explicitly specified should be computed.
- Possible entries in the data association for a given type include:
-
"Entities" association giving values of properties for each entity "EntityClasses" association giving entities and properties for entity classes "Label" label to use in displaying this type "LabelPlural" plural form of label to use in displaying this type "Properties" association giving information on properties for this type "PropertyClasses" association giving property class information for this type "EntityTypes" association giving properties and values for child types - The "Properties" entry in data is of the form <"propa"-><k1->v1,…>,"propb"->…,… >. Possible entries in the association given for each property include:
-
"DefaultFunction" default function to compute the value of this property "Label" label to use in displaying this property - With the setting "DefaultFunction"->f for a given property, f is applied to an entity to compute the value of that property if it is not specified explicitly in the "Entities" association.
- Possible entries for each entity class in "EntityClasses" include:
-
"Entities" list of names or predicates specifying entities in the class "Label" label to use in displaying this entity class - In the association for a particular entity class, "Entities"->{"name1","name2",…} gives an explicit list of entities in the entity class. "Entities"->"prop"->pred specifies that the entities in the class are those for which pred gives True when applied to the value of the property prop.
- Possible entries for each property class in "PropertyClasses" include:
-
"Label" label to use in displaying this property class "Properties" names of properties in this property class - The "EntityTypes" element of data gives information about child types of a given entity type. A child type consists of a subset of entities of that type—called its parent type—to which additional properties apply.
- The "EntityTypes" element of data is of the form <"child1"data1,… >, where datai corresponding to child type "childi" has the same form as data.
- Child types inherit entities from their parent types. Properties, entity classes and property classes of a child type "child" of a type "type" have the form EntityProperty["type""child",…], EntityClass["type""child",…] and EntityPropertyClass["type""child",…]. More deeply nested types have the form "type"…"grandchild".
- In the form EntityStore[{"type1"->meta1,"type2"->meta2,…},databasespec], the metai can have the following forms:
-
{"col1","col2",…} a list of column names to be exposed {"prop1""col1",…} "coli" is renamed to "propi" {…,"propi"EntityFunction[…],…} "propi" is computed from an EntityFunction class the whole type is constructed from a computed class of entities - In the form EntityStore[{…,"typei"->class,…},…], class can be any of the heads: EntityClass, FilteredEntityClass, ExtendedEntityClass, SampledEntityClass, SortedEntityClass, AggregatedEntityClass, CombinedEntityClass.
- In relational-databases-backed entity stores, the structure of the first argument of EntityStore has the form <"Types"-><"type1"-><… >,"type2"-><… >,… > >, where each association on the right-hand side of each "typei" can have the following keys:
-
"Properties" an association defining the properties "EntityTypeExtractor" a table name or a computed class "CanonicalNameProperties" the properties that form the CanonicalName - In relational databases, properties come in three flavors: columns on the original database, computed properties or relations.
- Column properties are defined with an association containing:
-
"ColumnPrefix" typically, the name of the table "ColumnName" the name of the column - Computed properties are defined with an association containing:
-
"Function" an EntityFunction defining the property - Relations properties are properties that take Entity or EntityClass values referencing types in the EntityStore; they are defined by an association containing:
-
"DestinationEntityType" the entity type referenced by the relation "EntityTypeMapping" the mapping between the properties of the incoming and destination entity type - "EntityTypeMapping" has the same specification as CombinedEntityClass and can take values of the form:
-
"prop" the origin and destination types have the same values for the property "prop" {"prop1","prop2",…} the origin and destination types have the same values for all "propi" "left""right" the origin type has values for "left" that are the same as the values for "right" in the destination type {"left1""right1",…} the origin type value of "lefti" is the same as the destination type value for "righti" EntityFunction[{left,right},…] the value of the relation for the origin entity left is all the destination type entities right for which the entity function evaluates to True - In relational-database-backed entity stores, formatting using "Label" properties is disabled to ensure that all calls to EntityValue and EntityList perform a single call to the database.
- The following options can be given:
-
Initialization None an expression to evaluate when the entity store is registered MetaInformation < > metainformation associatedwith the entity store
Examples
open allclose allBasic Examples (3)
Create an entity store for entities with type "fruit":
Register this entity store in the global list of entity stores:
Now property values for an entity are automatically looked up in the entity store:
Get the list of entities of the specified type:
Find entities whose value of the property "color" is Red:
Get an association of all entities and values for a specified property:
To connect to an external database, construct a RelationalDatabase object:
Construct an EntityStore from the RelationalDatabase object:
Register the EntityStore:
Construct a database-backed EntityStore with a subset of the original tables in the database:
Scope (11)
Basic Uses (4)
Create an entity store with a computed property "p3" and entity and property classes:
Get an Association of the value of all properties in "pc1" for one entity:
Access the entity store directly:
Access properties of an entity class:
Retrieve the raw data stored for "Entities":
Create an entity store of all integers:
Get the numerical value of an entity:
Compute the factorial of the entity "4":
Invalid entities are rejected:
Change the value of the "Factorial" of the entity "7":
This introduces an entity together with the new value into the registered entity store for "integer":
Other entities do not have an explicit value:
Define an EntityStore containing labeled entities and entity classes:
Normally an EntityClass behaves like the list of entities it represents. Get the "Label" for each entity in the class:
To get the "Label" of the class, access the EntityStore directly:
Put an entity store into the cloud:
Share the cloud object with someone who can then use the entities contained in it:
Database Connectivity (5)
Construct an EntityStore renaming the tables:
Construct an EntityStore with a subset of the columns:
Construct an EntityStore renaming the columns:
Construct an EntityStore with computed types:
Construct an EntityStore with computed properties:
Qualifiers (1)
Specify "beehive" entities with a "Date"-qualified "Population" property:
Get the latest population for Lisa's beehive:
Get the population for mid-2014:
Use Dated:
Child Types (1)
Create an entity store with people, some of whom are musicians or scientists:
List properties applicable to all people:
Concrete entities can have additional properties:
Get all data for an entity, including data from child types:
When there is no ambiguity, the property of a child type can be specified as a string:
The following uses a complete specification of a child type's property:
List all people working in the field of physics:
Options (2)
Possible Issues (2)
In database-backed entity stores, "Label" properties are not used for formatting:
When dealing with external databases, one might encounter a database where the primary key was not set for some tables:

When this happens, EntityStore will emit a message. This means that some functionality involving single entities will be disabled:

But EntityValue will generally keep working:
The only way around this problem is to set the primary key constraint in the external database.
Text
Wolfram Research (2016), EntityStore, Wolfram Language function, https://reference.wolfram.com/language/ref/EntityStore.html (updated 2019).
CMS
Wolfram Language. 2016. "EntityStore." Wolfram Language & System Documentation Center. Wolfram Research. Last Modified 2019. https://reference.wolfram.com/language/ref/EntityStore.html.
APA
Wolfram Language. (2016). EntityStore. Wolfram Language & System Documentation Center. Retrieved from https://reference.wolfram.com/language/ref/EntityStore.html