"Counter" (Data Structure)
"Counter"
represents a mutable integer counter.
Details
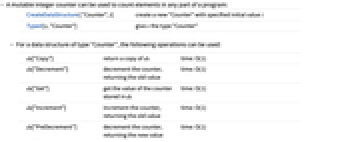
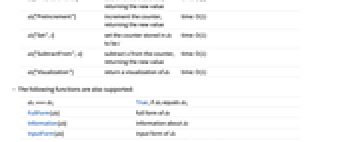
- A mutable integer counter can be used to count elements in any part of a program:
-
CreateDataStructure[ "Counter",i] create a new "Counter" with specified initial value i Typed[x,"Counter"] give x the type "Counter" - For a data structure of type "Counter", the following operations can be used:
-
ds["AddTo",n] add n to the counter, returning the new value time: O(1) ds["Copy"] return a copy of ds time: O(1) ds["Decrement"] decrement the counter, returning the old value time: O(1) ds["Get"] get the value of the counter stored in ds time: O(1) ds["Increment"] increment the counter, returning the old value time: O(1) ds["PreDecrement"] decrement the counter, returning the new value time: O(1) ds["PreIncrement"] increment the counter, returning the new value time: O(1) ds["Set",i] set the counter stored in ds to be i time: O(1) ds["SubtractFrom",n] subtract n from the counter, returning the new value time: O(1) ds["Visualization"] return a visualization of ds time: O(1) - The following functions are also supported:
-
dsi===dsj True, if dsi equals dsj FullForm[ds] full form of ds Information[ds] information about ds InputForm[ds] input form of ds Normal[ds] convert ds to a normal expression
Examples
open allclose allBasic Examples (1)
A new "Counter" can be created with CreateDataStructure:
Increment the value stored in the counter:
Confirm that the value has updated:
Scope (2)
Mutability (1)
Create a new "Counter" data structure and initialize it:
Define a function that increments the counter if its argument is even:
Information (1)
A new "Counter" can be created with CreateDataStructure:
Properties & Relations (1)
Create a new "Counter" data structure and initialize it:
Incrementing the counter returns the old counter value:
Pre-incrementing the counter returns the current counter value:
Setting the counter returns the current counter value: