"DynamicArray" (Data Structure)
"DynamicArray"
represents a dynamically extensible array where the elements are general expressions.
Details
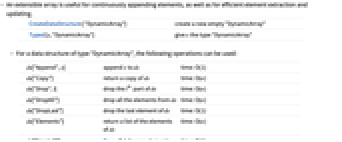
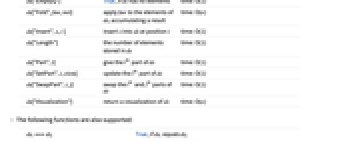
- An extensible array is useful for continuously appending elements, as well as for efficient element extraction and updating.
-
CreateDataStructure[ "DynamicArray"] create a new empty "DynamicArray" CreateDataStructure[ "DynamicArray",elems] create a new "DynamicArray" containing elems Typed[x,"DynamicArray"] give x the type "DynamicArray" - For a data structure of type "DynamicArray", the following operations can be used:
-
ds["Append",x] append x to ds time: O(1) ds["Copy"] return a copy of ds time: O(n) ds["Drop",i] drop the i part of ds
time: O(n) ds["DropAll"] drop all the elements from ds time: O(n) ds["DropLast"] drop the last element of ds time: O(1) ds["Elements"] return a list of the elements of ds time: O(n) ds["EmptyQ"] True, if ds has no elements time: O(1) ds["Fold",fun,init] apply fun to the elements of ds, starting with init, accumulating a result time: O(n) ds["Insert",x,i ] insert x into ds at position i time: O(1) ds["JoinBack",elems ] join elems to the back of ds time: O(nelems) ds["Length"] the number of elements stored in ds time: O(1) ds["Part",i] give the i part of ds
time: O(1) ds["SetPart",i,elem] update the i part of ds
time: O(1) ds["SwapPart",i,j] swap the i and j
parts of ds
time: O(1) ds["Visualization"] return a visualization of ds time: O(n) - The following functions are also supported:
-
dsi===dsj True, if dsi equals dsj ds["Part",i]=val set i element of ds to val
FullForm[ds] full form of ds Information[ds] information about ds InputForm[ds] input form of ds Normal[ds] convert ds to a normal expression
Examples
open allclose allBasic Examples (2)
A new "DynamicArray" can be created with CreateDataStructure:
Return an expression version of ds:
Scope (1)
Information (1)
A new "DynamicArray" can be created with CreateDataStructure:
Applications (11)
Reverse (1)
Bubble Sort (1)
Insertion Sort (1)
Quicksort (1)
Merge Sort (1)
Heapsort (1)
Timsort (1)
Fisher–Yates Shuffle (1)
Palindrome (1)
Quickselect (1)
Heap (1)
A heap is a tree-based data structure where the value stored at children is smaller than that stored in their parents (for a min heap). It is commonly used to implement a priority queue and typically implemented with an array rather than a tree.
The following converts an array into a heap:
A sample data structure to use:
This converts the array into a heap; it is hard to see the heap property:
This function generates a tree:
A visualization makes it easier to see the min heap property:
Properties & Relations (1)
"FixedArray" (1)
Many algorithms that work for "DynamicArray" also work for "FixedArray".